How To Code A Flash Loan without collateral
So how can you code a flash loan with a few easy steps? And how can you interact with Aave’s smart contracts? You’ll find answers to these questions in this tutorial.
What are Flash Loans?
A flash loan is a feature that allows you to borrow any available amount of assets from a designated smart contract pool with no collateral. Flash loans are useful building blocks in DeFi as they can be used for things like arbitrage, swapping collateral and self-liquidation.
Flash loans, although initially introduced by the Marble protocol, were popularised by Aave and Dydx.
So, what’s the catch?
A flash loan has to be borrowed and repaid within the same blockchain transaction.
If you’d like to learn more about flash loans check out our article on flash loans here.
Before we start
This guide requires a basic understanding of Solidity smart contracts and how to use Remix. You can find Remix documentation here.
It also requires having your Metamask wallet installed and switched to the Kovan testnet.
To execute any smart contracts on Ethereum you’ll also have to have some ETH in your wallet. You can get some Kovan testnet ETH by going to faucet.kovan.network and logging in with your Github account. You can request 1 testnet ETH once per day.
We’ll also need some DAI. To request DAI go to testnet.aave.com/faucet and click on DAI, than hit the “Submit” button.
Okay, the last step is to set up your Remix environment. Go to remix.ethereum.org and select 0.6.6+ version of the Solidity compiler.
Flash Loan Smart Contract
Great, time to start working on our flash loan. Let’s start with creating a basic smart contract file.
Here is the first piece of code we need.
This will import all the necessary dependencies and create a basic FlashLoan.sol smart contract that inherits from FlashLoanReceiverBase which is an abstract contract that provides a few useful things such as a way of repaying the flash loan.
The Flashloan.sol constructor accepts the address of one of the Aave’s lending pool providers. We’ll get to this later.
You can now hit the compile button.
This should download all the relevant dependencies and result in an error as we haven’t implemented all of the necessary methods from the FlashLoanReceiverBase just yet.
Time to add 2 missing functions. First one is our actual flashLoan function that will be used to trigger a flash loan. The other one is the missing method from FlashLoanReceiverBase – executeOperation that will be called after the flash loan method is triggered.
Flash Loan Function
Let’s add the flashLoan function first.
Here is the code snippet.
Time to make sure we understand all the relevant parts.
The flashLoan function parameter _asset is an address of an asset that we want to borrow using a flash loan, for example, ETH or DAI.
We define our function as onlyOwner, so only the owner of the contract can call the function.
uint amount = 1000000000000000000;
Here, we’re defining the borrowed amount in the minor unit – wei that is 10^18, so this value is equal to 1. If we pass the DAI address as _asset we’ll be borrowing 1 DAI, if we pass the ETH address we’ll be borrowing 1 ETH.
Now we can use a ILendingPool interface provided by Aave and call flashLoan function with all the required parameters such as the asset that we want to borrow, the amount of that asset and an extra data parameter.
Even after adding our flashLoan function, the code will still not compile as we have to add the missing executeOperation function.
executeOperation Function
Time to implement the last missing bit before we can try triggering our flash loan function.
executeOperation function will be called by the LendingPool contract after a valid amount of asset is requested in a flash loan.
Here is a code snippet for the executeOperation function.
All the parameters for the executeOperation function will be passed automatically after a valid flash loan is triggered using the flashLoan function.
The require check is used to validate that we received a correct amount from a flash loan.
Next, we can insert any arbitrary logic that we want to execute. At this step we have all the funds from a flash loan available, so we can, for example, try to execute an arbitrage opportunity.
After we made use of a flash loan, it’s time to repay it.
uint totalDebt = _amount.add(_fee);
The last step is to call transferFundsBackToPoolInternal to pay back our flash loan.
Now, we can try to compile our code again. This time everything should compile just fine.
Time to trigger our flashLoan function.
Running The Code
Great, our flash loan smart contract is compiled and ready to be deployed.
Let’s start with preparing 2 necessary things:
- LendingPoolAddressesProvider – to deploy our smart contract we need to find the address of the Aave’s lending smart contract on the Kovan testnet. You can find all the addresses here. The Kovan address is 0x506B0B2CF20FAA8f38a4E2B524EE43e1f4458Cc5
- DAI address – we need a contract address of DAI (or any other asset you want to borrow) on the Kovan testnet. The DAI Kovan address is 0xFf795577d9AC8bD7D90Ee22b6C1703490b6512FD
Time to deploy our smart contract.
- Go to “Deploy & Run Transactions” on Remix
- Select “Injected Web3” (Make sure your Metamask is switched to Kovan)
- Select a contract to be deployed – FlashLoan.sol
- Pass the LendingPoolAddressesProvider that we found a few steps earlier to the field next to the “Deploy” button
- Hit “Deploy” button
- Confirm your Ethereum transaction via Metamask
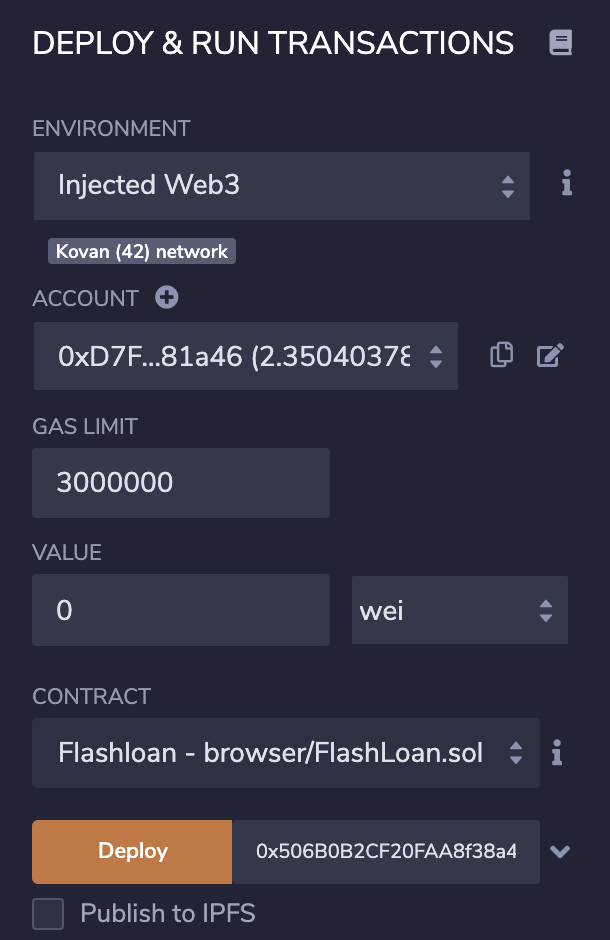
If everything went ok – great! We now have our FlashLoan.sol smart contract deployed on the Ethereum testnet – Kovan.
Here is the crucial step in running our smart contract. We have to send some DAI into the smart contract we just created to be able to pay for the flash loan fee.
You can find your smart contract address by checking the Metamask transaction that you previously approved. The transaction for creating a smart contract should look similar to this one here. From that transaction, you can extract the smart contract address (0x27016b23BEE0553A4aAa89b25Be58b93Fe647BBe in our case).
Time to trigger our flashLoan function from the deployed contract. Remember about passing a correct address of the asset you want to borrow. In our case it’s DAI address on the Kovan testnet.
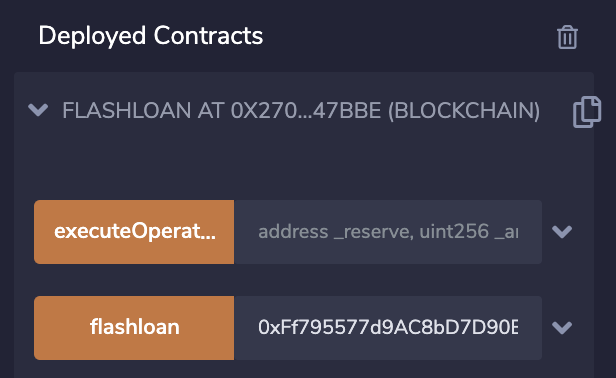
After triggering the flashLoan function and accepting an Ethereum transaction via Metamask, you should see a similar transaction to this one here.
Congrats! You just made your first flash loan!
If you enjoyed this tutorial you can also check out Finematics on Youtube and Twitter.
Comments
Post a Comment